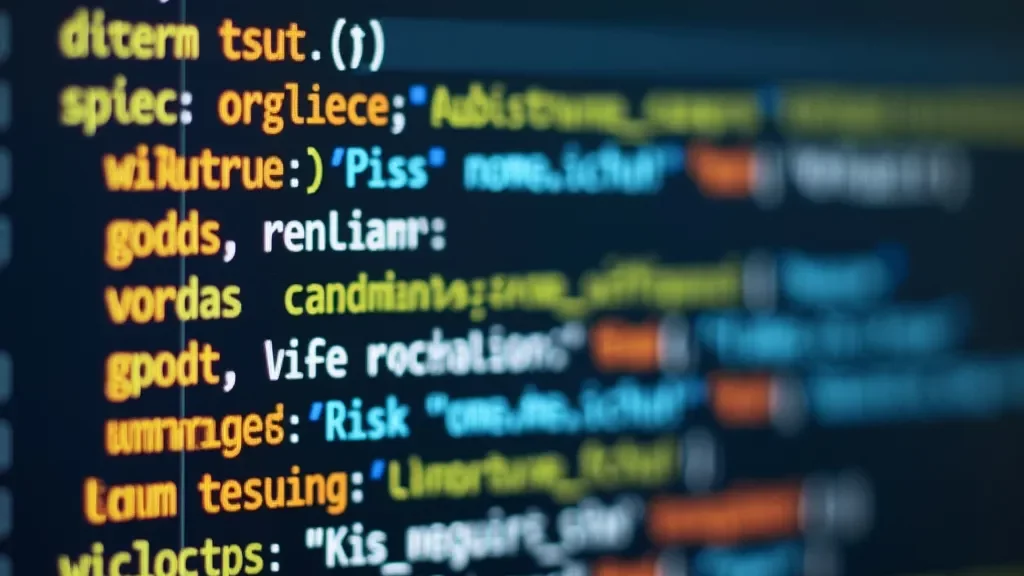
Best Practices for Writing Testable Code
In the ever-evolving landscape of software development, the ability to write testable code is paramount. Testable code not only enhances the reliability of applications but also facilitates easier maintenance and collaboration among developers. Here are some best practices to help you write code that is easy to test.
1. Follow the Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class or module should only have one reason to change. By adhering to this principle, you can ensure that your code is modular, making it easier to write unit tests for individual components. Each class or function should focus on a specific task, reducing complexity and dependencies.
Example:
Instead of having a class that handles both user authentication and data storage, separate these concerns into two distinct classes. This separation makes it easier to test each component independently.
2. Write Small Functions
Smaller functions are easier to understand, debug, and test. Aim for functions that perform a single task and have a limited number of parameters. This approach reduces the cognitive load when writing tests and makes it straightforward to determine expected outcomes.
Example:
Instead of writing a long function that processes user data, break it down into smaller functions that handle validation, transformation, and storage separately.
3. Use Dependency Injection
Dependency injection is a design pattern that allows a class to receive its dependencies from an external source rather than creating them internally. This practice promotes loose coupling and makes it easier to substitute mock objects during testing.
Example:
Instead of hardcoding a database connection within your class, pass the connection as a parameter. This strategy allows you to inject a mock connection during testing.
4. Embrace Interfaces and Abstract Classes
Using interfaces and abstract classes can help decouple your code, making it more flexible and easier to test. By programming against abstractions rather than concrete implementations, you can easily swap out dependencies for testing purposes.
Example:
Define an interface for payment processing. This way, you can create mock implementations for testing various scenarios without relying on the actual payment processor.
5. Keep State Mutable and Controlled
When writing code that maintains state, ensure that the state is mutable and controlled through well-defined interfaces. This approach prevents unintended side effects and allows for easier tracking of changes during testing.
Example:
Instead of relying on global state, encapsulate state within objects and expose methods that modify it. This encapsulation helps maintain control over how and when state changes occur.
6. Write Automated Tests First (TDD)
Test-Driven Development (TDD) emphasizes writing tests before writing the actual code. This practice helps clarify requirements and leads to the development of more testable code. By thinking about how you will test your code upfront, you can design it with testability in mind.
Example:
Start by writing a failing test case for a new feature. Then, write the minimum code necessary to pass the test, followed by refactoring the code while keeping the tests green.
7. Utilize Mocks and Stubs
Mocks and stubs are essential tools for testing. They allow you to simulate the behavior of complex dependencies, making it easier to test components in isolation. Use them liberally to verify interactions and control the environment in which your tests run.
Example:
When testing a service that relies on an external API, use a mock API client to simulate various responses and error conditions without making actual network calls.
8. Consistent Naming Conventions
Consistent naming conventions improve code readability and maintainability. When naming functions, classes, and variables, opt for clear and descriptive names that reflect their purpose. This clarity helps other developers (and your future self) understand the code’s intent, making it easier to write tests.
Example:
Instead of using vague names like handleData
, use validateUserData
or processPayment
, which clearly convey the function’s purpose.
9. Document Your Code
Documentation is vital for maintaining testable code. Clearly document the purpose and expected behavior of your functions and classes. This practice helps other developers understand how to test your code effectively and reduces ambiguity.
Example:
Include comments about the expected input and output of functions, as well as any side effects they may have.
Conclusion
Writing testable code is a skill that pays dividends in the long run. By following these best practices, you can create software that is not only robust and reliable but also easier to maintain and extend. Emphasizing modularity, clarity, and simplicity will lead to a codebase that stands the test of time, making both development and testing a more productive and enjoyable experience.