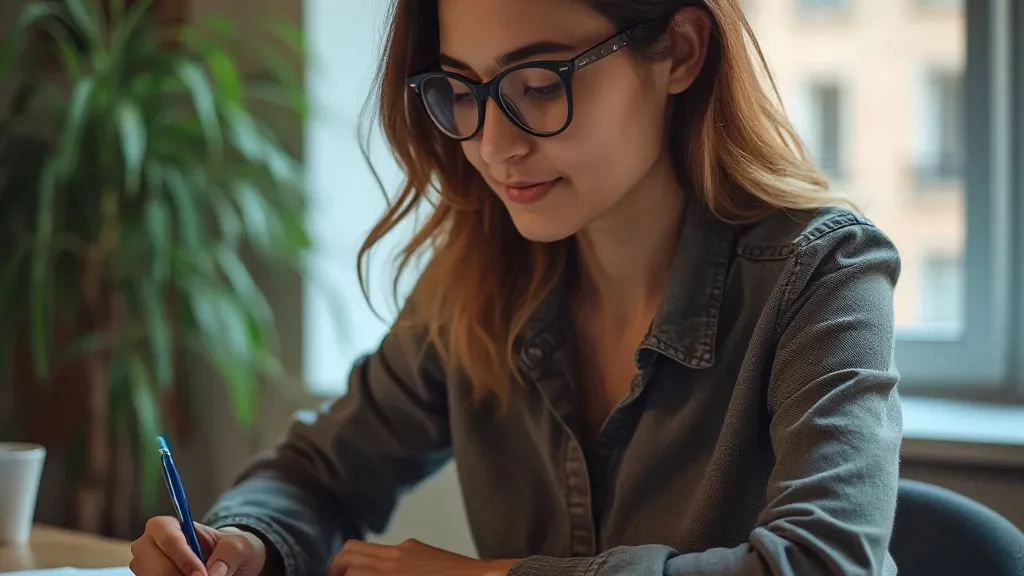
The Importance of Comprehensive Software Documentation
In the world of software development, documentation often takes a back seat to coding and feature implementation. However, well-documented software is critical for long-term maintainability, ease of onboarding new developers, and ensuring that the project can adapt and evolve over time. This article explores the best practices for documenting software effectively, emphasizing the importance of self-explanatory code, architectural clarity, and the integration of testing as part of the documentation process.
1. Writing Self-Explanatory Code
The cornerstone of effective documentation begins with the code itself. Code should be written in a manner that is easily understandable. This means using descriptive variable names, clear function names, and following consistent naming conventions. By doing this, the code becomes self-documenting to a significant extent, allowing developers to grasp its purpose without extensive external explanations.
Best Practices for Self-Explanatory Code:
- Descriptive Naming: Use names that convey the purpose of variables and functions. For example, instead of naming a variable
x
, useuserAge
ortotalPrice
. - Clear Structure: Organize code logically with a clean structure, using functions and classes to encapsulate functionality.
- Minimal Comments: Only include comments for complex algorithms, unusual business rules, or technical details that are not immediately clear from the code itself.
2. Documenting Technical and Business Rules
While the majority of your code should be self-explanatory, there will always be unique business rules or technical specifications that may require additional clarification. Comments should be used sparingly and strategically to explain these complex parts of the code.
Examples of When to Use Comments:
- Clarifying Business Logic: If a piece of code implements an unusual business requirement, document its rationale and implications.
- Technical Notes: For specific algorithms or methods that are not standard, a brief explanation will help future developers understand why they were implemented in a particular way.
3. Architectural Documentation
A comprehensive overview of the software architecture should be included in your documentation. This page should provide an understanding of how different components interact and the technologies used in the project.
Key Elements of Architectural Documentation:
- System Overview: Provide a high-level description of the architecture, including diagrams if possible.
- Links to Services: Include references to external services, frameworks, or libraries that are part of the architecture. For instance, if your application interacts with AWS services, list them with links to their documentation.
Example:
### Architecture Overview
Our application follows a microservices architecture, enabling scalability and maintainability.
- **Service A:** Handles user authentication (Link to [Auth0](https://auth0.com))
- **Service B:** Manages product inventory (Link to [Firebase](https://firebase.google.com))
4. Development Environment Setup
A dedicated page for setting up the development environment is essential. This section should guide new developers through the installation process and ensure that they can get up and running quickly.
Steps to Install the Development Environment:
- Clone the Repository: Provide the command to clone the project repository.
- Install Dependencies: List the necessary dependencies and commands for installation. Include version information if applicable.
- Environment Variables: Outline any required environment variables and how to set them up.
Example:
### Setting Up the Development Environment
1. Clone the repository:
git clone https://github.com/your-repo.git
2. Install dependencies:
npm install
3. Set environment variables:
- `DATABASE_URL`: URL for the database connection
- `API_KEY`: Key for external API access
5. Integrating Testing as Documentation
Unit tests and integration tests are not just for ensuring code quality; they also serve as an essential part of documentation. Well-written tests provide examples of how the code is meant to be used and expected behaviors.
Documentation Through Testing:
- Unit Tests: Ensure that functions and components behave as intended. Each test case can act as a usage example.
- Integration Tests: Document the interactions between systems and components, providing insight into how different parts of the application work together.
Example:
describe('Calculator', () => {
it('should add two numbers', () => {
expect(add(2, 3)).toBe(5);
});
});
Integration Testing
Integration tests validate the interaction between the authentication service and the database:
describe('Authentication Integration', () => {
it('should create a new user and store in the database', async () => {
const user = await createUser({ username: 'testUser' });
expect(user).toBeDefined();
// Further checks with the database...
});
});
Conclusion
Effective software documentation is not merely an afterthought; it is an integral part of the development process. By emphasizing self-explanatory code, providing clear architectural documentation, guiding developers through setup, and treating tests as documentation, teams can create a robust framework that supports ongoing development and maintenance. This approach not only enhances the immediate usability of the code but also ensures its longevity and adaptability in the future.